forked from ROMEO/nexosim
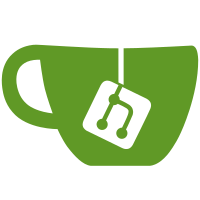
TODO: return the list of models involved in a deadlock. Note that Many execution errors are not implemented at all at the moment and will need separate PRs, namely: - Terminated - ModelError - Panic
1069 lines
47 KiB
Rust
1069 lines
47 KiB
Rust
// This file is @generated by prost-build.
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct Error {
|
|
#[prost(enumeration = "ErrorCode", tag = "1")]
|
|
pub code: i32,
|
|
#[prost(string, tag = "2")]
|
|
pub message: ::prost::alloc::string::String,
|
|
}
|
|
#[derive(Clone, Copy, PartialEq, ::prost::Message)]
|
|
pub struct EventKey {
|
|
#[prost(uint64, tag = "1")]
|
|
pub subkey1: u64,
|
|
#[prost(uint64, tag = "2")]
|
|
pub subkey2: u64,
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct InitRequest {
|
|
#[prost(message, optional, tag = "1")]
|
|
pub time: ::core::option::Option<::prost_types::Timestamp>,
|
|
#[prost(bytes = "vec", tag = "2")]
|
|
pub cfg: ::prost::alloc::vec::Vec<u8>,
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct InitReply {
|
|
/// Always returns exactly 1 variant.
|
|
#[prost(oneof = "init_reply::Result", tags = "1, 100")]
|
|
pub result: ::core::option::Option<init_reply::Result>,
|
|
}
|
|
/// Nested message and enum types in `InitReply`.
|
|
pub mod init_reply {
|
|
/// Always returns exactly 1 variant.
|
|
#[derive(Clone, PartialEq, ::prost::Oneof)]
|
|
pub enum Result {
|
|
#[prost(message, tag = "1")]
|
|
Empty(()),
|
|
#[prost(message, tag = "100")]
|
|
Error(super::Error),
|
|
}
|
|
}
|
|
#[derive(Clone, Copy, PartialEq, ::prost::Message)]
|
|
pub struct TimeRequest {}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct TimeReply {
|
|
/// Always returns exactly 1 variant.
|
|
#[prost(oneof = "time_reply::Result", tags = "1, 100")]
|
|
pub result: ::core::option::Option<time_reply::Result>,
|
|
}
|
|
/// Nested message and enum types in `TimeReply`.
|
|
pub mod time_reply {
|
|
/// Always returns exactly 1 variant.
|
|
#[derive(Clone, PartialEq, ::prost::Oneof)]
|
|
pub enum Result {
|
|
#[prost(message, tag = "1")]
|
|
Time(::prost_types::Timestamp),
|
|
#[prost(message, tag = "100")]
|
|
Error(super::Error),
|
|
}
|
|
}
|
|
#[derive(Clone, Copy, PartialEq, ::prost::Message)]
|
|
pub struct StepRequest {}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct StepReply {
|
|
/// Always returns exactly 1 variant.
|
|
#[prost(oneof = "step_reply::Result", tags = "1, 100")]
|
|
pub result: ::core::option::Option<step_reply::Result>,
|
|
}
|
|
/// Nested message and enum types in `StepReply`.
|
|
pub mod step_reply {
|
|
/// Always returns exactly 1 variant.
|
|
#[derive(Clone, PartialEq, ::prost::Oneof)]
|
|
pub enum Result {
|
|
#[prost(message, tag = "1")]
|
|
Time(::prost_types::Timestamp),
|
|
#[prost(message, tag = "100")]
|
|
Error(super::Error),
|
|
}
|
|
}
|
|
#[derive(Clone, Copy, PartialEq, ::prost::Message)]
|
|
pub struct StepUntilRequest {
|
|
/// Always returns exactly 1 variant.
|
|
#[prost(oneof = "step_until_request::Deadline", tags = "1, 2")]
|
|
pub deadline: ::core::option::Option<step_until_request::Deadline>,
|
|
}
|
|
/// Nested message and enum types in `StepUntilRequest`.
|
|
pub mod step_until_request {
|
|
/// Always returns exactly 1 variant.
|
|
#[derive(Clone, Copy, PartialEq, ::prost::Oneof)]
|
|
pub enum Deadline {
|
|
#[prost(message, tag = "1")]
|
|
Time(::prost_types::Timestamp),
|
|
#[prost(message, tag = "2")]
|
|
Duration(::prost_types::Duration),
|
|
}
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct StepUntilReply {
|
|
/// Always returns exactly 1 variant.
|
|
#[prost(oneof = "step_until_reply::Result", tags = "1, 100")]
|
|
pub result: ::core::option::Option<step_until_reply::Result>,
|
|
}
|
|
/// Nested message and enum types in `StepUntilReply`.
|
|
pub mod step_until_reply {
|
|
/// Always returns exactly 1 variant.
|
|
#[derive(Clone, PartialEq, ::prost::Oneof)]
|
|
pub enum Result {
|
|
#[prost(message, tag = "1")]
|
|
Time(::prost_types::Timestamp),
|
|
#[prost(message, tag = "100")]
|
|
Error(super::Error),
|
|
}
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct ScheduleEventRequest {
|
|
#[prost(string, tag = "3")]
|
|
pub source_name: ::prost::alloc::string::String,
|
|
#[prost(bytes = "vec", tag = "4")]
|
|
pub event: ::prost::alloc::vec::Vec<u8>,
|
|
#[prost(message, optional, tag = "5")]
|
|
pub period: ::core::option::Option<::prost_types::Duration>,
|
|
#[prost(bool, tag = "6")]
|
|
pub with_key: bool,
|
|
/// Expects exactly 1 variant.
|
|
#[prost(oneof = "schedule_event_request::Deadline", tags = "1, 2")]
|
|
pub deadline: ::core::option::Option<schedule_event_request::Deadline>,
|
|
}
|
|
/// Nested message and enum types in `ScheduleEventRequest`.
|
|
pub mod schedule_event_request {
|
|
/// Expects exactly 1 variant.
|
|
#[derive(Clone, Copy, PartialEq, ::prost::Oneof)]
|
|
pub enum Deadline {
|
|
#[prost(message, tag = "1")]
|
|
Time(::prost_types::Timestamp),
|
|
#[prost(message, tag = "2")]
|
|
Duration(::prost_types::Duration),
|
|
}
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct ScheduleEventReply {
|
|
/// Always returns exactly 1 variant.
|
|
#[prost(oneof = "schedule_event_reply::Result", tags = "1, 2, 100")]
|
|
pub result: ::core::option::Option<schedule_event_reply::Result>,
|
|
}
|
|
/// Nested message and enum types in `ScheduleEventReply`.
|
|
pub mod schedule_event_reply {
|
|
/// Always returns exactly 1 variant.
|
|
#[derive(Clone, PartialEq, ::prost::Oneof)]
|
|
pub enum Result {
|
|
#[prost(message, tag = "1")]
|
|
Empty(()),
|
|
#[prost(message, tag = "2")]
|
|
Key(super::EventKey),
|
|
#[prost(message, tag = "100")]
|
|
Error(super::Error),
|
|
}
|
|
}
|
|
#[derive(Clone, Copy, PartialEq, ::prost::Message)]
|
|
pub struct CancelEventRequest {
|
|
#[prost(message, optional, tag = "1")]
|
|
pub key: ::core::option::Option<EventKey>,
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct CancelEventReply {
|
|
/// Always returns exactly 1 variant.
|
|
#[prost(oneof = "cancel_event_reply::Result", tags = "1, 100")]
|
|
pub result: ::core::option::Option<cancel_event_reply::Result>,
|
|
}
|
|
/// Nested message and enum types in `CancelEventReply`.
|
|
pub mod cancel_event_reply {
|
|
/// Always returns exactly 1 variant.
|
|
#[derive(Clone, PartialEq, ::prost::Oneof)]
|
|
pub enum Result {
|
|
#[prost(message, tag = "1")]
|
|
Empty(()),
|
|
#[prost(message, tag = "100")]
|
|
Error(super::Error),
|
|
}
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct ProcessEventRequest {
|
|
#[prost(string, tag = "1")]
|
|
pub source_name: ::prost::alloc::string::String,
|
|
#[prost(bytes = "vec", tag = "2")]
|
|
pub event: ::prost::alloc::vec::Vec<u8>,
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct ProcessEventReply {
|
|
/// Always returns exactly 1 variant.
|
|
#[prost(oneof = "process_event_reply::Result", tags = "1, 100")]
|
|
pub result: ::core::option::Option<process_event_reply::Result>,
|
|
}
|
|
/// Nested message and enum types in `ProcessEventReply`.
|
|
pub mod process_event_reply {
|
|
/// Always returns exactly 1 variant.
|
|
#[derive(Clone, PartialEq, ::prost::Oneof)]
|
|
pub enum Result {
|
|
#[prost(message, tag = "1")]
|
|
Empty(()),
|
|
#[prost(message, tag = "100")]
|
|
Error(super::Error),
|
|
}
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct ProcessQueryRequest {
|
|
#[prost(string, tag = "1")]
|
|
pub source_name: ::prost::alloc::string::String,
|
|
#[prost(bytes = "vec", tag = "2")]
|
|
pub request: ::prost::alloc::vec::Vec<u8>,
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct ProcessQueryReply {
|
|
/// This field is hoisted because protobuf3 does not support `repeated` within
|
|
/// a `oneof`. It is Always empty if an error is returned
|
|
#[prost(bytes = "vec", repeated, tag = "1")]
|
|
pub replies: ::prost::alloc::vec::Vec<::prost::alloc::vec::Vec<u8>>,
|
|
/// Always returns exactly 1 variant.
|
|
#[prost(oneof = "process_query_reply::Result", tags = "10, 100")]
|
|
pub result: ::core::option::Option<process_query_reply::Result>,
|
|
}
|
|
/// Nested message and enum types in `ProcessQueryReply`.
|
|
pub mod process_query_reply {
|
|
/// Always returns exactly 1 variant.
|
|
#[derive(Clone, PartialEq, ::prost::Oneof)]
|
|
pub enum Result {
|
|
#[prost(message, tag = "10")]
|
|
Empty(()),
|
|
#[prost(message, tag = "100")]
|
|
Error(super::Error),
|
|
}
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct ReadEventsRequest {
|
|
#[prost(string, tag = "1")]
|
|
pub sink_name: ::prost::alloc::string::String,
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct ReadEventsReply {
|
|
/// This field is hoisted because protobuf3 does not support `repeated` within
|
|
/// a `oneof`. It is Always empty if an error is returned
|
|
#[prost(bytes = "vec", repeated, tag = "1")]
|
|
pub events: ::prost::alloc::vec::Vec<::prost::alloc::vec::Vec<u8>>,
|
|
/// Always returns exactly 1 variant.
|
|
#[prost(oneof = "read_events_reply::Result", tags = "10, 100")]
|
|
pub result: ::core::option::Option<read_events_reply::Result>,
|
|
}
|
|
/// Nested message and enum types in `ReadEventsReply`.
|
|
pub mod read_events_reply {
|
|
/// Always returns exactly 1 variant.
|
|
#[derive(Clone, PartialEq, ::prost::Oneof)]
|
|
pub enum Result {
|
|
#[prost(message, tag = "10")]
|
|
Empty(()),
|
|
#[prost(message, tag = "100")]
|
|
Error(super::Error),
|
|
}
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct OpenSinkRequest {
|
|
#[prost(string, tag = "1")]
|
|
pub sink_name: ::prost::alloc::string::String,
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct OpenSinkReply {
|
|
/// Always returns exactly 1 variant.
|
|
#[prost(oneof = "open_sink_reply::Result", tags = "10, 100")]
|
|
pub result: ::core::option::Option<open_sink_reply::Result>,
|
|
}
|
|
/// Nested message and enum types in `OpenSinkReply`.
|
|
pub mod open_sink_reply {
|
|
/// Always returns exactly 1 variant.
|
|
#[derive(Clone, PartialEq, ::prost::Oneof)]
|
|
pub enum Result {
|
|
#[prost(message, tag = "10")]
|
|
Empty(()),
|
|
#[prost(message, tag = "100")]
|
|
Error(super::Error),
|
|
}
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct CloseSinkRequest {
|
|
#[prost(string, tag = "1")]
|
|
pub sink_name: ::prost::alloc::string::String,
|
|
}
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct CloseSinkReply {
|
|
/// Always returns exactly 1 variant.
|
|
#[prost(oneof = "close_sink_reply::Result", tags = "10, 100")]
|
|
pub result: ::core::option::Option<close_sink_reply::Result>,
|
|
}
|
|
/// Nested message and enum types in `CloseSinkReply`.
|
|
pub mod close_sink_reply {
|
|
/// Always returns exactly 1 variant.
|
|
#[derive(Clone, PartialEq, ::prost::Oneof)]
|
|
pub enum Result {
|
|
#[prost(message, tag = "10")]
|
|
Empty(()),
|
|
#[prost(message, tag = "100")]
|
|
Error(super::Error),
|
|
}
|
|
}
|
|
/// A convenience message type for custom transport implementation.
|
|
#[derive(Clone, PartialEq, ::prost::Message)]
|
|
pub struct AnyRequest {
|
|
/// Expects exactly 1 variant.
|
|
#[prost(oneof = "any_request::Request", tags = "1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11")]
|
|
pub request: ::core::option::Option<any_request::Request>,
|
|
}
|
|
/// Nested message and enum types in `AnyRequest`.
|
|
pub mod any_request {
|
|
/// Expects exactly 1 variant.
|
|
#[derive(Clone, PartialEq, ::prost::Oneof)]
|
|
pub enum Request {
|
|
#[prost(message, tag = "1")]
|
|
InitRequest(super::InitRequest),
|
|
#[prost(message, tag = "2")]
|
|
TimeRequest(super::TimeRequest),
|
|
#[prost(message, tag = "3")]
|
|
StepRequest(super::StepRequest),
|
|
#[prost(message, tag = "4")]
|
|
StepUntilRequest(super::StepUntilRequest),
|
|
#[prost(message, tag = "5")]
|
|
ScheduleEventRequest(super::ScheduleEventRequest),
|
|
#[prost(message, tag = "6")]
|
|
CancelEventRequest(super::CancelEventRequest),
|
|
#[prost(message, tag = "7")]
|
|
ProcessEventRequest(super::ProcessEventRequest),
|
|
#[prost(message, tag = "8")]
|
|
ProcessQueryRequest(super::ProcessQueryRequest),
|
|
#[prost(message, tag = "9")]
|
|
ReadEventsRequest(super::ReadEventsRequest),
|
|
#[prost(message, tag = "10")]
|
|
OpenSinkRequest(super::OpenSinkRequest),
|
|
#[prost(message, tag = "11")]
|
|
CloseSinkRequest(super::CloseSinkRequest),
|
|
}
|
|
}
|
|
#[derive(Clone, Copy, Debug, PartialEq, Eq, Hash, PartialOrd, Ord, ::prost::Enumeration)]
|
|
#[repr(i32)]
|
|
pub enum ErrorCode {
|
|
InternalError = 0,
|
|
SimulationNotStarted = 1,
|
|
SimulationTerminated = 2,
|
|
SimulationDeadlock = 3,
|
|
SimulationModelError = 4,
|
|
SimulationPanic = 5,
|
|
SimulationBadQuery = 6,
|
|
SimulationTimeOutOfRange = 22,
|
|
MissingArgument = 7,
|
|
InvalidTime = 8,
|
|
InvalidDuration = 9,
|
|
InvalidPeriod = 10,
|
|
InvalidMessage = 11,
|
|
InvalidKey = 12,
|
|
SourceNotFound = 20,
|
|
SinkNotFound = 21,
|
|
}
|
|
impl ErrorCode {
|
|
/// String value of the enum field names used in the ProtoBuf definition.
|
|
///
|
|
/// The values are not transformed in any way and thus are considered stable
|
|
/// (if the ProtoBuf definition does not change) and safe for programmatic use.
|
|
pub fn as_str_name(&self) -> &'static str {
|
|
match self {
|
|
ErrorCode::InternalError => "INTERNAL_ERROR",
|
|
ErrorCode::SimulationNotStarted => "SIMULATION_NOT_STARTED",
|
|
ErrorCode::SimulationTerminated => "SIMULATION_TERMINATED",
|
|
ErrorCode::SimulationDeadlock => "SIMULATION_DEADLOCK",
|
|
ErrorCode::SimulationModelError => "SIMULATION_MODEL_ERROR",
|
|
ErrorCode::SimulationPanic => "SIMULATION_PANIC",
|
|
ErrorCode::SimulationBadQuery => "SIMULATION_BAD_QUERY",
|
|
ErrorCode::SimulationTimeOutOfRange => "SIMULATION_TIME_OUT_OF_RANGE",
|
|
ErrorCode::MissingArgument => "MISSING_ARGUMENT",
|
|
ErrorCode::InvalidTime => "INVALID_TIME",
|
|
ErrorCode::InvalidDuration => "INVALID_DURATION",
|
|
ErrorCode::InvalidPeriod => "INVALID_PERIOD",
|
|
ErrorCode::InvalidMessage => "INVALID_MESSAGE",
|
|
ErrorCode::InvalidKey => "INVALID_KEY",
|
|
ErrorCode::SourceNotFound => "SOURCE_NOT_FOUND",
|
|
ErrorCode::SinkNotFound => "SINK_NOT_FOUND",
|
|
}
|
|
}
|
|
/// Creates an enum from field names used in the ProtoBuf definition.
|
|
pub fn from_str_name(value: &str) -> ::core::option::Option<Self> {
|
|
match value {
|
|
"INTERNAL_ERROR" => Some(Self::InternalError),
|
|
"SIMULATION_NOT_STARTED" => Some(Self::SimulationNotStarted),
|
|
"SIMULATION_TERMINATED" => Some(Self::SimulationTerminated),
|
|
"SIMULATION_DEADLOCK" => Some(Self::SimulationDeadlock),
|
|
"SIMULATION_MODEL_ERROR" => Some(Self::SimulationModelError),
|
|
"SIMULATION_PANIC" => Some(Self::SimulationPanic),
|
|
"SIMULATION_BAD_QUERY" => Some(Self::SimulationBadQuery),
|
|
"SIMULATION_TIME_OUT_OF_RANGE" => Some(Self::SimulationTimeOutOfRange),
|
|
"MISSING_ARGUMENT" => Some(Self::MissingArgument),
|
|
"INVALID_TIME" => Some(Self::InvalidTime),
|
|
"INVALID_DURATION" => Some(Self::InvalidDuration),
|
|
"INVALID_PERIOD" => Some(Self::InvalidPeriod),
|
|
"INVALID_MESSAGE" => Some(Self::InvalidMessage),
|
|
"INVALID_KEY" => Some(Self::InvalidKey),
|
|
"SOURCE_NOT_FOUND" => Some(Self::SourceNotFound),
|
|
"SINK_NOT_FOUND" => Some(Self::SinkNotFound),
|
|
_ => None,
|
|
}
|
|
}
|
|
}
|
|
/// Generated server implementations.
|
|
pub mod simulation_server {
|
|
#![allow(unused_variables, dead_code, missing_docs, clippy::let_unit_value)]
|
|
use tonic::codegen::*;
|
|
/// Generated trait containing gRPC methods that should be implemented for use with SimulationServer.
|
|
#[async_trait]
|
|
pub trait Simulation: std::marker::Send + std::marker::Sync + 'static {
|
|
async fn init(
|
|
&self,
|
|
request: tonic::Request<super::InitRequest>,
|
|
) -> std::result::Result<tonic::Response<super::InitReply>, tonic::Status>;
|
|
async fn time(
|
|
&self,
|
|
request: tonic::Request<super::TimeRequest>,
|
|
) -> std::result::Result<tonic::Response<super::TimeReply>, tonic::Status>;
|
|
async fn step(
|
|
&self,
|
|
request: tonic::Request<super::StepRequest>,
|
|
) -> std::result::Result<tonic::Response<super::StepReply>, tonic::Status>;
|
|
async fn step_until(
|
|
&self,
|
|
request: tonic::Request<super::StepUntilRequest>,
|
|
) -> std::result::Result<tonic::Response<super::StepUntilReply>, tonic::Status>;
|
|
async fn schedule_event(
|
|
&self,
|
|
request: tonic::Request<super::ScheduleEventRequest>,
|
|
) -> std::result::Result<
|
|
tonic::Response<super::ScheduleEventReply>,
|
|
tonic::Status,
|
|
>;
|
|
async fn cancel_event(
|
|
&self,
|
|
request: tonic::Request<super::CancelEventRequest>,
|
|
) -> std::result::Result<
|
|
tonic::Response<super::CancelEventReply>,
|
|
tonic::Status,
|
|
>;
|
|
async fn process_event(
|
|
&self,
|
|
request: tonic::Request<super::ProcessEventRequest>,
|
|
) -> std::result::Result<
|
|
tonic::Response<super::ProcessEventReply>,
|
|
tonic::Status,
|
|
>;
|
|
async fn process_query(
|
|
&self,
|
|
request: tonic::Request<super::ProcessQueryRequest>,
|
|
) -> std::result::Result<
|
|
tonic::Response<super::ProcessQueryReply>,
|
|
tonic::Status,
|
|
>;
|
|
async fn read_events(
|
|
&self,
|
|
request: tonic::Request<super::ReadEventsRequest>,
|
|
) -> std::result::Result<tonic::Response<super::ReadEventsReply>, tonic::Status>;
|
|
async fn open_sink(
|
|
&self,
|
|
request: tonic::Request<super::OpenSinkRequest>,
|
|
) -> std::result::Result<tonic::Response<super::OpenSinkReply>, tonic::Status>;
|
|
async fn close_sink(
|
|
&self,
|
|
request: tonic::Request<super::CloseSinkRequest>,
|
|
) -> std::result::Result<tonic::Response<super::CloseSinkReply>, tonic::Status>;
|
|
}
|
|
#[derive(Debug)]
|
|
pub struct SimulationServer<T> {
|
|
inner: Arc<T>,
|
|
accept_compression_encodings: EnabledCompressionEncodings,
|
|
send_compression_encodings: EnabledCompressionEncodings,
|
|
max_decoding_message_size: Option<usize>,
|
|
max_encoding_message_size: Option<usize>,
|
|
}
|
|
impl<T> SimulationServer<T> {
|
|
pub fn new(inner: T) -> Self {
|
|
Self::from_arc(Arc::new(inner))
|
|
}
|
|
pub fn from_arc(inner: Arc<T>) -> Self {
|
|
Self {
|
|
inner,
|
|
accept_compression_encodings: Default::default(),
|
|
send_compression_encodings: Default::default(),
|
|
max_decoding_message_size: None,
|
|
max_encoding_message_size: None,
|
|
}
|
|
}
|
|
pub fn with_interceptor<F>(
|
|
inner: T,
|
|
interceptor: F,
|
|
) -> InterceptedService<Self, F>
|
|
where
|
|
F: tonic::service::Interceptor,
|
|
{
|
|
InterceptedService::new(Self::new(inner), interceptor)
|
|
}
|
|
/// Enable decompressing requests with the given encoding.
|
|
#[must_use]
|
|
pub fn accept_compressed(mut self, encoding: CompressionEncoding) -> Self {
|
|
self.accept_compression_encodings.enable(encoding);
|
|
self
|
|
}
|
|
/// Compress responses with the given encoding, if the client supports it.
|
|
#[must_use]
|
|
pub fn send_compressed(mut self, encoding: CompressionEncoding) -> Self {
|
|
self.send_compression_encodings.enable(encoding);
|
|
self
|
|
}
|
|
/// Limits the maximum size of a decoded message.
|
|
///
|
|
/// Default: `4MB`
|
|
#[must_use]
|
|
pub fn max_decoding_message_size(mut self, limit: usize) -> Self {
|
|
self.max_decoding_message_size = Some(limit);
|
|
self
|
|
}
|
|
/// Limits the maximum size of an encoded message.
|
|
///
|
|
/// Default: `usize::MAX`
|
|
#[must_use]
|
|
pub fn max_encoding_message_size(mut self, limit: usize) -> Self {
|
|
self.max_encoding_message_size = Some(limit);
|
|
self
|
|
}
|
|
}
|
|
impl<T, B> tonic::codegen::Service<http::Request<B>> for SimulationServer<T>
|
|
where
|
|
T: Simulation,
|
|
B: Body + std::marker::Send + 'static,
|
|
B::Error: Into<StdError> + std::marker::Send + 'static,
|
|
{
|
|
type Response = http::Response<tonic::body::BoxBody>;
|
|
type Error = std::convert::Infallible;
|
|
type Future = BoxFuture<Self::Response, Self::Error>;
|
|
fn poll_ready(
|
|
&mut self,
|
|
_cx: &mut Context<'_>,
|
|
) -> Poll<std::result::Result<(), Self::Error>> {
|
|
Poll::Ready(Ok(()))
|
|
}
|
|
fn call(&mut self, req: http::Request<B>) -> Self::Future {
|
|
match req.uri().path() {
|
|
"/simulation.Simulation/Init" => {
|
|
#[allow(non_camel_case_types)]
|
|
struct InitSvc<T: Simulation>(pub Arc<T>);
|
|
impl<T: Simulation> tonic::server::UnaryService<super::InitRequest>
|
|
for InitSvc<T> {
|
|
type Response = super::InitReply;
|
|
type Future = BoxFuture<
|
|
tonic::Response<Self::Response>,
|
|
tonic::Status,
|
|
>;
|
|
fn call(
|
|
&mut self,
|
|
request: tonic::Request<super::InitRequest>,
|
|
) -> Self::Future {
|
|
let inner = Arc::clone(&self.0);
|
|
let fut = async move {
|
|
<T as Simulation>::init(&inner, request).await
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
}
|
|
let accept_compression_encodings = self.accept_compression_encodings;
|
|
let send_compression_encodings = self.send_compression_encodings;
|
|
let max_decoding_message_size = self.max_decoding_message_size;
|
|
let max_encoding_message_size = self.max_encoding_message_size;
|
|
let inner = self.inner.clone();
|
|
let fut = async move {
|
|
let method = InitSvc(inner);
|
|
let codec = tonic::codec::ProstCodec::default();
|
|
let mut grpc = tonic::server::Grpc::new(codec)
|
|
.apply_compression_config(
|
|
accept_compression_encodings,
|
|
send_compression_encodings,
|
|
)
|
|
.apply_max_message_size_config(
|
|
max_decoding_message_size,
|
|
max_encoding_message_size,
|
|
);
|
|
let res = grpc.unary(method, req).await;
|
|
Ok(res)
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
"/simulation.Simulation/Time" => {
|
|
#[allow(non_camel_case_types)]
|
|
struct TimeSvc<T: Simulation>(pub Arc<T>);
|
|
impl<T: Simulation> tonic::server::UnaryService<super::TimeRequest>
|
|
for TimeSvc<T> {
|
|
type Response = super::TimeReply;
|
|
type Future = BoxFuture<
|
|
tonic::Response<Self::Response>,
|
|
tonic::Status,
|
|
>;
|
|
fn call(
|
|
&mut self,
|
|
request: tonic::Request<super::TimeRequest>,
|
|
) -> Self::Future {
|
|
let inner = Arc::clone(&self.0);
|
|
let fut = async move {
|
|
<T as Simulation>::time(&inner, request).await
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
}
|
|
let accept_compression_encodings = self.accept_compression_encodings;
|
|
let send_compression_encodings = self.send_compression_encodings;
|
|
let max_decoding_message_size = self.max_decoding_message_size;
|
|
let max_encoding_message_size = self.max_encoding_message_size;
|
|
let inner = self.inner.clone();
|
|
let fut = async move {
|
|
let method = TimeSvc(inner);
|
|
let codec = tonic::codec::ProstCodec::default();
|
|
let mut grpc = tonic::server::Grpc::new(codec)
|
|
.apply_compression_config(
|
|
accept_compression_encodings,
|
|
send_compression_encodings,
|
|
)
|
|
.apply_max_message_size_config(
|
|
max_decoding_message_size,
|
|
max_encoding_message_size,
|
|
);
|
|
let res = grpc.unary(method, req).await;
|
|
Ok(res)
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
"/simulation.Simulation/Step" => {
|
|
#[allow(non_camel_case_types)]
|
|
struct StepSvc<T: Simulation>(pub Arc<T>);
|
|
impl<T: Simulation> tonic::server::UnaryService<super::StepRequest>
|
|
for StepSvc<T> {
|
|
type Response = super::StepReply;
|
|
type Future = BoxFuture<
|
|
tonic::Response<Self::Response>,
|
|
tonic::Status,
|
|
>;
|
|
fn call(
|
|
&mut self,
|
|
request: tonic::Request<super::StepRequest>,
|
|
) -> Self::Future {
|
|
let inner = Arc::clone(&self.0);
|
|
let fut = async move {
|
|
<T as Simulation>::step(&inner, request).await
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
}
|
|
let accept_compression_encodings = self.accept_compression_encodings;
|
|
let send_compression_encodings = self.send_compression_encodings;
|
|
let max_decoding_message_size = self.max_decoding_message_size;
|
|
let max_encoding_message_size = self.max_encoding_message_size;
|
|
let inner = self.inner.clone();
|
|
let fut = async move {
|
|
let method = StepSvc(inner);
|
|
let codec = tonic::codec::ProstCodec::default();
|
|
let mut grpc = tonic::server::Grpc::new(codec)
|
|
.apply_compression_config(
|
|
accept_compression_encodings,
|
|
send_compression_encodings,
|
|
)
|
|
.apply_max_message_size_config(
|
|
max_decoding_message_size,
|
|
max_encoding_message_size,
|
|
);
|
|
let res = grpc.unary(method, req).await;
|
|
Ok(res)
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
"/simulation.Simulation/StepUntil" => {
|
|
#[allow(non_camel_case_types)]
|
|
struct StepUntilSvc<T: Simulation>(pub Arc<T>);
|
|
impl<
|
|
T: Simulation,
|
|
> tonic::server::UnaryService<super::StepUntilRequest>
|
|
for StepUntilSvc<T> {
|
|
type Response = super::StepUntilReply;
|
|
type Future = BoxFuture<
|
|
tonic::Response<Self::Response>,
|
|
tonic::Status,
|
|
>;
|
|
fn call(
|
|
&mut self,
|
|
request: tonic::Request<super::StepUntilRequest>,
|
|
) -> Self::Future {
|
|
let inner = Arc::clone(&self.0);
|
|
let fut = async move {
|
|
<T as Simulation>::step_until(&inner, request).await
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
}
|
|
let accept_compression_encodings = self.accept_compression_encodings;
|
|
let send_compression_encodings = self.send_compression_encodings;
|
|
let max_decoding_message_size = self.max_decoding_message_size;
|
|
let max_encoding_message_size = self.max_encoding_message_size;
|
|
let inner = self.inner.clone();
|
|
let fut = async move {
|
|
let method = StepUntilSvc(inner);
|
|
let codec = tonic::codec::ProstCodec::default();
|
|
let mut grpc = tonic::server::Grpc::new(codec)
|
|
.apply_compression_config(
|
|
accept_compression_encodings,
|
|
send_compression_encodings,
|
|
)
|
|
.apply_max_message_size_config(
|
|
max_decoding_message_size,
|
|
max_encoding_message_size,
|
|
);
|
|
let res = grpc.unary(method, req).await;
|
|
Ok(res)
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
"/simulation.Simulation/ScheduleEvent" => {
|
|
#[allow(non_camel_case_types)]
|
|
struct ScheduleEventSvc<T: Simulation>(pub Arc<T>);
|
|
impl<
|
|
T: Simulation,
|
|
> tonic::server::UnaryService<super::ScheduleEventRequest>
|
|
for ScheduleEventSvc<T> {
|
|
type Response = super::ScheduleEventReply;
|
|
type Future = BoxFuture<
|
|
tonic::Response<Self::Response>,
|
|
tonic::Status,
|
|
>;
|
|
fn call(
|
|
&mut self,
|
|
request: tonic::Request<super::ScheduleEventRequest>,
|
|
) -> Self::Future {
|
|
let inner = Arc::clone(&self.0);
|
|
let fut = async move {
|
|
<T as Simulation>::schedule_event(&inner, request).await
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
}
|
|
let accept_compression_encodings = self.accept_compression_encodings;
|
|
let send_compression_encodings = self.send_compression_encodings;
|
|
let max_decoding_message_size = self.max_decoding_message_size;
|
|
let max_encoding_message_size = self.max_encoding_message_size;
|
|
let inner = self.inner.clone();
|
|
let fut = async move {
|
|
let method = ScheduleEventSvc(inner);
|
|
let codec = tonic::codec::ProstCodec::default();
|
|
let mut grpc = tonic::server::Grpc::new(codec)
|
|
.apply_compression_config(
|
|
accept_compression_encodings,
|
|
send_compression_encodings,
|
|
)
|
|
.apply_max_message_size_config(
|
|
max_decoding_message_size,
|
|
max_encoding_message_size,
|
|
);
|
|
let res = grpc.unary(method, req).await;
|
|
Ok(res)
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
"/simulation.Simulation/CancelEvent" => {
|
|
#[allow(non_camel_case_types)]
|
|
struct CancelEventSvc<T: Simulation>(pub Arc<T>);
|
|
impl<
|
|
T: Simulation,
|
|
> tonic::server::UnaryService<super::CancelEventRequest>
|
|
for CancelEventSvc<T> {
|
|
type Response = super::CancelEventReply;
|
|
type Future = BoxFuture<
|
|
tonic::Response<Self::Response>,
|
|
tonic::Status,
|
|
>;
|
|
fn call(
|
|
&mut self,
|
|
request: tonic::Request<super::CancelEventRequest>,
|
|
) -> Self::Future {
|
|
let inner = Arc::clone(&self.0);
|
|
let fut = async move {
|
|
<T as Simulation>::cancel_event(&inner, request).await
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
}
|
|
let accept_compression_encodings = self.accept_compression_encodings;
|
|
let send_compression_encodings = self.send_compression_encodings;
|
|
let max_decoding_message_size = self.max_decoding_message_size;
|
|
let max_encoding_message_size = self.max_encoding_message_size;
|
|
let inner = self.inner.clone();
|
|
let fut = async move {
|
|
let method = CancelEventSvc(inner);
|
|
let codec = tonic::codec::ProstCodec::default();
|
|
let mut grpc = tonic::server::Grpc::new(codec)
|
|
.apply_compression_config(
|
|
accept_compression_encodings,
|
|
send_compression_encodings,
|
|
)
|
|
.apply_max_message_size_config(
|
|
max_decoding_message_size,
|
|
max_encoding_message_size,
|
|
);
|
|
let res = grpc.unary(method, req).await;
|
|
Ok(res)
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
"/simulation.Simulation/ProcessEvent" => {
|
|
#[allow(non_camel_case_types)]
|
|
struct ProcessEventSvc<T: Simulation>(pub Arc<T>);
|
|
impl<
|
|
T: Simulation,
|
|
> tonic::server::UnaryService<super::ProcessEventRequest>
|
|
for ProcessEventSvc<T> {
|
|
type Response = super::ProcessEventReply;
|
|
type Future = BoxFuture<
|
|
tonic::Response<Self::Response>,
|
|
tonic::Status,
|
|
>;
|
|
fn call(
|
|
&mut self,
|
|
request: tonic::Request<super::ProcessEventRequest>,
|
|
) -> Self::Future {
|
|
let inner = Arc::clone(&self.0);
|
|
let fut = async move {
|
|
<T as Simulation>::process_event(&inner, request).await
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
}
|
|
let accept_compression_encodings = self.accept_compression_encodings;
|
|
let send_compression_encodings = self.send_compression_encodings;
|
|
let max_decoding_message_size = self.max_decoding_message_size;
|
|
let max_encoding_message_size = self.max_encoding_message_size;
|
|
let inner = self.inner.clone();
|
|
let fut = async move {
|
|
let method = ProcessEventSvc(inner);
|
|
let codec = tonic::codec::ProstCodec::default();
|
|
let mut grpc = tonic::server::Grpc::new(codec)
|
|
.apply_compression_config(
|
|
accept_compression_encodings,
|
|
send_compression_encodings,
|
|
)
|
|
.apply_max_message_size_config(
|
|
max_decoding_message_size,
|
|
max_encoding_message_size,
|
|
);
|
|
let res = grpc.unary(method, req).await;
|
|
Ok(res)
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
"/simulation.Simulation/ProcessQuery" => {
|
|
#[allow(non_camel_case_types)]
|
|
struct ProcessQuerySvc<T: Simulation>(pub Arc<T>);
|
|
impl<
|
|
T: Simulation,
|
|
> tonic::server::UnaryService<super::ProcessQueryRequest>
|
|
for ProcessQuerySvc<T> {
|
|
type Response = super::ProcessQueryReply;
|
|
type Future = BoxFuture<
|
|
tonic::Response<Self::Response>,
|
|
tonic::Status,
|
|
>;
|
|
fn call(
|
|
&mut self,
|
|
request: tonic::Request<super::ProcessQueryRequest>,
|
|
) -> Self::Future {
|
|
let inner = Arc::clone(&self.0);
|
|
let fut = async move {
|
|
<T as Simulation>::process_query(&inner, request).await
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
}
|
|
let accept_compression_encodings = self.accept_compression_encodings;
|
|
let send_compression_encodings = self.send_compression_encodings;
|
|
let max_decoding_message_size = self.max_decoding_message_size;
|
|
let max_encoding_message_size = self.max_encoding_message_size;
|
|
let inner = self.inner.clone();
|
|
let fut = async move {
|
|
let method = ProcessQuerySvc(inner);
|
|
let codec = tonic::codec::ProstCodec::default();
|
|
let mut grpc = tonic::server::Grpc::new(codec)
|
|
.apply_compression_config(
|
|
accept_compression_encodings,
|
|
send_compression_encodings,
|
|
)
|
|
.apply_max_message_size_config(
|
|
max_decoding_message_size,
|
|
max_encoding_message_size,
|
|
);
|
|
let res = grpc.unary(method, req).await;
|
|
Ok(res)
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
"/simulation.Simulation/ReadEvents" => {
|
|
#[allow(non_camel_case_types)]
|
|
struct ReadEventsSvc<T: Simulation>(pub Arc<T>);
|
|
impl<
|
|
T: Simulation,
|
|
> tonic::server::UnaryService<super::ReadEventsRequest>
|
|
for ReadEventsSvc<T> {
|
|
type Response = super::ReadEventsReply;
|
|
type Future = BoxFuture<
|
|
tonic::Response<Self::Response>,
|
|
tonic::Status,
|
|
>;
|
|
fn call(
|
|
&mut self,
|
|
request: tonic::Request<super::ReadEventsRequest>,
|
|
) -> Self::Future {
|
|
let inner = Arc::clone(&self.0);
|
|
let fut = async move {
|
|
<T as Simulation>::read_events(&inner, request).await
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
}
|
|
let accept_compression_encodings = self.accept_compression_encodings;
|
|
let send_compression_encodings = self.send_compression_encodings;
|
|
let max_decoding_message_size = self.max_decoding_message_size;
|
|
let max_encoding_message_size = self.max_encoding_message_size;
|
|
let inner = self.inner.clone();
|
|
let fut = async move {
|
|
let method = ReadEventsSvc(inner);
|
|
let codec = tonic::codec::ProstCodec::default();
|
|
let mut grpc = tonic::server::Grpc::new(codec)
|
|
.apply_compression_config(
|
|
accept_compression_encodings,
|
|
send_compression_encodings,
|
|
)
|
|
.apply_max_message_size_config(
|
|
max_decoding_message_size,
|
|
max_encoding_message_size,
|
|
);
|
|
let res = grpc.unary(method, req).await;
|
|
Ok(res)
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
"/simulation.Simulation/OpenSink" => {
|
|
#[allow(non_camel_case_types)]
|
|
struct OpenSinkSvc<T: Simulation>(pub Arc<T>);
|
|
impl<
|
|
T: Simulation,
|
|
> tonic::server::UnaryService<super::OpenSinkRequest>
|
|
for OpenSinkSvc<T> {
|
|
type Response = super::OpenSinkReply;
|
|
type Future = BoxFuture<
|
|
tonic::Response<Self::Response>,
|
|
tonic::Status,
|
|
>;
|
|
fn call(
|
|
&mut self,
|
|
request: tonic::Request<super::OpenSinkRequest>,
|
|
) -> Self::Future {
|
|
let inner = Arc::clone(&self.0);
|
|
let fut = async move {
|
|
<T as Simulation>::open_sink(&inner, request).await
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
}
|
|
let accept_compression_encodings = self.accept_compression_encodings;
|
|
let send_compression_encodings = self.send_compression_encodings;
|
|
let max_decoding_message_size = self.max_decoding_message_size;
|
|
let max_encoding_message_size = self.max_encoding_message_size;
|
|
let inner = self.inner.clone();
|
|
let fut = async move {
|
|
let method = OpenSinkSvc(inner);
|
|
let codec = tonic::codec::ProstCodec::default();
|
|
let mut grpc = tonic::server::Grpc::new(codec)
|
|
.apply_compression_config(
|
|
accept_compression_encodings,
|
|
send_compression_encodings,
|
|
)
|
|
.apply_max_message_size_config(
|
|
max_decoding_message_size,
|
|
max_encoding_message_size,
|
|
);
|
|
let res = grpc.unary(method, req).await;
|
|
Ok(res)
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
"/simulation.Simulation/CloseSink" => {
|
|
#[allow(non_camel_case_types)]
|
|
struct CloseSinkSvc<T: Simulation>(pub Arc<T>);
|
|
impl<
|
|
T: Simulation,
|
|
> tonic::server::UnaryService<super::CloseSinkRequest>
|
|
for CloseSinkSvc<T> {
|
|
type Response = super::CloseSinkReply;
|
|
type Future = BoxFuture<
|
|
tonic::Response<Self::Response>,
|
|
tonic::Status,
|
|
>;
|
|
fn call(
|
|
&mut self,
|
|
request: tonic::Request<super::CloseSinkRequest>,
|
|
) -> Self::Future {
|
|
let inner = Arc::clone(&self.0);
|
|
let fut = async move {
|
|
<T as Simulation>::close_sink(&inner, request).await
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
}
|
|
let accept_compression_encodings = self.accept_compression_encodings;
|
|
let send_compression_encodings = self.send_compression_encodings;
|
|
let max_decoding_message_size = self.max_decoding_message_size;
|
|
let max_encoding_message_size = self.max_encoding_message_size;
|
|
let inner = self.inner.clone();
|
|
let fut = async move {
|
|
let method = CloseSinkSvc(inner);
|
|
let codec = tonic::codec::ProstCodec::default();
|
|
let mut grpc = tonic::server::Grpc::new(codec)
|
|
.apply_compression_config(
|
|
accept_compression_encodings,
|
|
send_compression_encodings,
|
|
)
|
|
.apply_max_message_size_config(
|
|
max_decoding_message_size,
|
|
max_encoding_message_size,
|
|
);
|
|
let res = grpc.unary(method, req).await;
|
|
Ok(res)
|
|
};
|
|
Box::pin(fut)
|
|
}
|
|
_ => {
|
|
Box::pin(async move {
|
|
Ok(
|
|
http::Response::builder()
|
|
.status(200)
|
|
.header("grpc-status", tonic::Code::Unimplemented as i32)
|
|
.header(
|
|
http::header::CONTENT_TYPE,
|
|
tonic::metadata::GRPC_CONTENT_TYPE,
|
|
)
|
|
.body(empty_body())
|
|
.unwrap(),
|
|
)
|
|
})
|
|
}
|
|
}
|
|
}
|
|
}
|
|
impl<T> Clone for SimulationServer<T> {
|
|
fn clone(&self) -> Self {
|
|
let inner = self.inner.clone();
|
|
Self {
|
|
inner,
|
|
accept_compression_encodings: self.accept_compression_encodings,
|
|
send_compression_encodings: self.send_compression_encodings,
|
|
max_decoding_message_size: self.max_decoding_message_size,
|
|
max_encoding_message_size: self.max_encoding_message_size,
|
|
}
|
|
}
|
|
}
|
|
/// Generated gRPC service name
|
|
pub const SERVICE_NAME: &str = "simulation.Simulation";
|
|
impl<T> tonic::server::NamedService for SimulationServer<T> {
|
|
const NAME: &'static str = SERVICE_NAME;
|
|
}
|
|
}
|